2.2: Assignment Operators | Variables
Learning Objectives
By the end of this lesson, you should be able to:
Describe what a variable is and how to assign it a value or expression.
Use the
var
keyword to declare a new JavaScript variable.Explain how to achieve accurate representation of your program with suitable variable names.
Use the camelCase naming convention for your JavaScript variable names.
Introduction
To do more than just basic math, we need to store, access and manipulate data in a computer's memory. We do this by utilizing variables in programming.
What are Variables?
Variables store and contain data. We name a variable and we associate a specific data value with it. We use variables to represent data that our program will process. First, we name a variable by using the keyword var
. This tells the browser that the next word is the intended name for the variable.
In order to assign a value to a variable, we use the assignment operator =
. In programming, the equals sign has a slightly different meaning than in a mathematical equation, we are using =
declaratively:
var pi = 3.14;
var radius = 4;
In the above statements we have declared the variables pi
and radius
, and assigned them the value 3.14
and 4
respectively. Using these 2 data values we can calculate the area of a circle.
pi * radius * radius;
We can also use variables to capture the result of that calculated value, and store it in memory.
var area = pi * radius * radius;
The Importance of Abstraction
If we wanted the same result we could also write the following.1var x = 3.14 * 4 * 4;Copied!But this would not be as meaningful as our previous example.Our programs must not only calculate values correctly, they must also accurately represent the operations we are performing, in this case calculating the area of a circle. The names of our variables give meaning to the data our code contains.
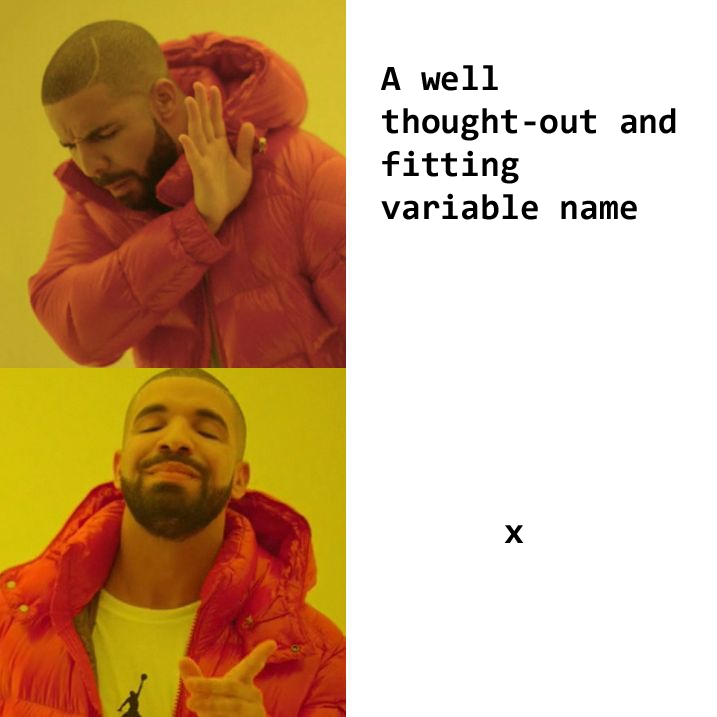
Variable Naming Convention
In JavaScript convention, ordinary variables are named with lower camel case. The name starts with a lowercase letter and every subsequent word starts with uppercase. For example, bananaCount
or firstDiceRoll
. This is convention, and will not affect code functionality. Different languages and communities have different conventions. Similar to driving conventions: in some countries, cars are driven on the left side, and on the right side in other countries.

Exercises
Using the Chrome Dev Tools Console, write code to represent each of the following. Use descriptive variable names that explain what you are calculating. You will need to search the web for some formulas. When you are done, paste the code into a message to yourself in the community channel.
Calculate the circumference of a circle given its radius
Convert Celsius to Fahrenheit
Calculate the volume of a cube given a side length
Calculate the gradient percentage of a road given rise and run
Convert millilitres to pints
Last updated